Setup MySQL
To check if MySQL is installed type
sudo mysql -v
This should output the version
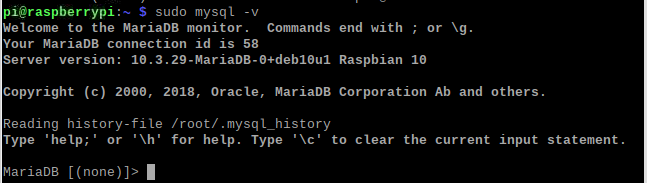
Create a database for the app:
Create a database to store results of CRUD operations.
CREATE DATABASE IF NOT EXISTS goappdb; USE goappdb;
Notes on Create Database
CREATE {DATABASE | SCHEMA} [IF NOT EXISTS] db_name [create_option] create_option: [DEFAULT] { CHARACTER SET [=] charset_name | COLLATE [=] collation_name | ENCRYPTION [=] {'Y' | 'N'} }
- CREATE DATABASE creates database with db_name (*Requires CREATE privileges, CREATE SCHEMA is a synonym for CREATE DATABASE,
LOCK TABLES
statement will not permit CREATE DATABASE) - CHARACTER SET option specifies the database character set
- COLLATE option specifies the default database collation
*The default MySQL server character set and collation are utf8mb4 and utf8mb4_0900_ai_ci - ENCRYPTION option defines the default database encryption, which is inherited by tables created in the database. The permitted values are ‘Y’ (encryption enabled) and ‘N’ (encryption disabled)
Next add a user for Go App
CREATE USER 'goApp'@'localhost' IDENTIFIED BY 'Pa$$w0rd'; GRANT ALL PRIVILEGES ON goappdb.* TO 'goApp'@'localhost'; FLUSH PRIVILEGES;
User Notes
Specific Privileges can be assigned using
GRANT type_of_permission ON database_name.table_name TO ‘username’@’localhost’;
type_of_permission:
- ALL PRIVILEGES- as we saw previously, this would allow a MySQL user full access to a designated database (or if no database is selected, global access across the system)
- CREATE- allows them to create new tables or databases
- DROP- allows them to them to delete tables or databases
- DELETE- allows them to delete rows from tables
- INSERT- allows them to insert rows into tables
- SELECT- allows them to use the
SELECT
command to read through databases - UPDATE- allow them to update table rows
- GRANT OPTION- allows them to grant or remove other users’ privileges
To remove permissions:
REVOKE type_of_permission ON database_name.table_name FROM ‘username’@’localhost’;
To remove a user:
DROP USER ‘username’@’localhost’;