Raspberry Pi GPIO Pins
Programming the GPIO pins on the Raspberry Pi using Python.
To Install Python on Rasbian
sudo apt-get install python3
Setup the circuit by connecting the positive end of a LED up to GPIO 4 (pin 7) and the negative end to a 330k ohm resistor and to a ground (pin 6,8, or any GND) similar to the diagram below.
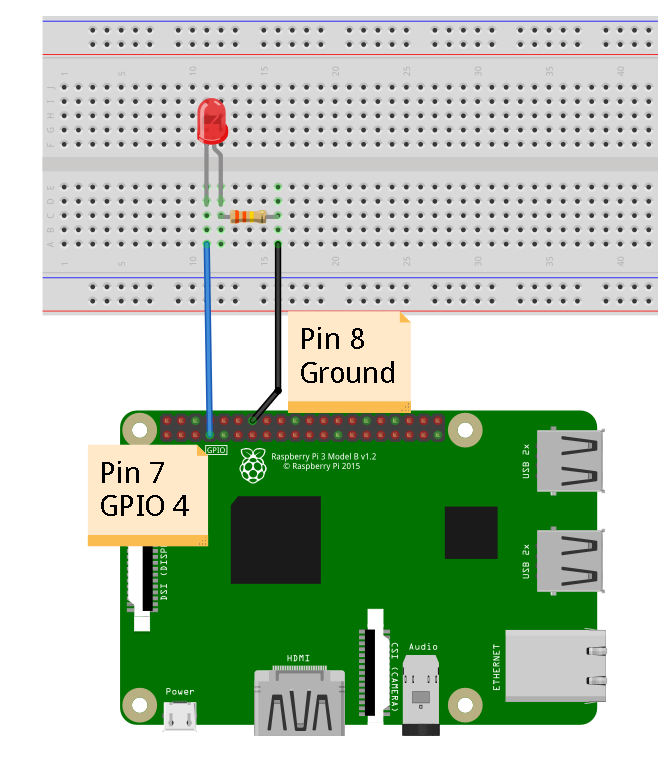
Create a python file
sudo nano led_blink.py
And insert the code:
#import the GPIO and time package import RPi.GPIO as GPIO import time GPIO.setmode(GPIO.BOARD) GPIO.setup(7, GPIO.OUT) # loop through 50 times, on/off for 1 second for i in range(50): GPIO.output(7,True) time.sleep(1) GPIO.output(7,False) time.sleep(1) GPIO.cleanup()
Now save and exit file. (Nano exit by pressing ctrl+x and then Answer yes to write to file by typing y)
Running the python script
Running the python script the circuit should power on the LED. The python script is run by executing the following command. It is also important to run it as the superuser using sudo.
sudo python led_blink.py
This concludes the GPIO pinout Tutorial.