Setting up visual studio MSDOCs
If using Visual Studio Code the C++ extension must be installed and a compiler must be installed on Windows OS. If Compiling For WSL Linux must have a compiler installed
MinGWx64 on Windows 10 via MSYS2,,
CLang on MacOs can be installed using XCODE
GNU compiler on Ubuntu/Debian WSL,
can be installed with command:
sudo apt-get install build-essential gdb
Creating C++ Work Folder
C++ Hello World
mkdir HelloWorld
cd HelloWorld
code .
Build Instructions
The tasks.json file list the instructions for VS Code to build(compile) the program into an executable file.
{ "tasks": [ { "type": "cppbuild", "label": "C/C++: g++.exe build active file", "command": "C:\\MinGW\\bin\\g++.exe", "args": [ "-g", "${file}", "-o", "${fileDirname}\\${fileBasenameNoExtension}.exe" ], "options": { "cwd": "${fileDirname}" }, "problemMatcher": [ "$gcc" ], "group": { "kind": "build", "isDefault": true }, "detail": "Task generated by Debugger." } ], "version": "2.0.0" }
- type
- label value is what you will see in the tasks list; you can name this whatever you like
- command setting specifies the program to run; in this case that is g++.
- args array specifies the command-line arguments that will be passed to g++. These arguments must be specified in the order expected by the compiler. This task tells g++ to take the active file (${file}), compile it, and create an executable file in the current directory (${fileDirname}) with the same name as the active file but with the .exe extension (${fileBasenameNoExtension}.exe), resulting in helloworld.exe for our example.
- options
- problemMatcher
- group
- kind
- “isDefault”: true value in the group object specifies that this task will be run when you press Ctrl+Shift+B. This property is for convenience only; if you set it to false, you can still run it from the Terminal menu with Tasks: Run Build Task.
- detail
Modifying tasks.json#
You can modify your tasks.json
to build multiple C++ files by using an argument like "${workspaceFolder}\\*.cpp"
instead of ${file}
. This will build all .cpp
files in your current folder. You can also modify the output filename by replacing "${fileDirname}\\${fileBasenameNoExtension}.exe"
with a hard-coded filename (for example "${workspaceFolder}\\myProgram.exe"
).
Add Hello World source code#
#include <iostream> using namespace std; int main() { cout << "Hello World" << endl; }
Save the File then Select the Terminal > Run Build Task command (Ctrl+Shift+B)
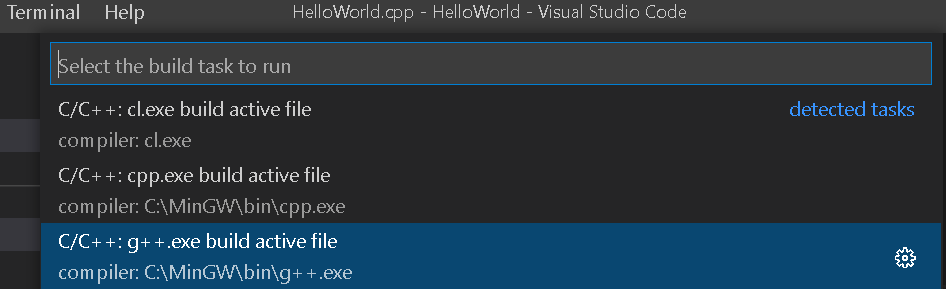
An executable file, HelloWorld.exe will be created and can be run to verify all steps are functioning.
Modify the HelloWorld.cpp file to a more elaborate Hello World
#include <iostream> #include <vector> #include <string> using namespace std; int main() { vector<string> msg {"Hello", "C++", "World", "from", "VS Code", "and the C++ extension!"}; for (const string& word : msg) { cout << word << " "; } cout << endl; }
Running the code . command will generate files:
tasks.json
(build instructions)launch.json
(debugger settings)c_cpp_properties.json
(compiler path and IntelliSense settings)
Setup Debugging
The instructions for debugging are listed in launch.json file, pressing F5 will launch the listed instructions. The file is generated when Select Run > Add Configuration… choose C++ (GDB/LLDB) and on the dropdown g++.exe build and debug active file is selected from the predefined configurations
{ "version": "0.2.0", "configurations": [ { "name": "g++.exe - Build and debug active file", "type": "cppdbg", "request": "launch", "program": "${fileDirname}\\${fileBasenameNoExtension}.exe", "args": [], "stopAtEntry": false, "cwd": "${fileDirname}", "environment": [], "externalConsole": false, "MIMode": "gdb", "miDebuggerPath": "C:\\MinGW\\bin\\gdb.exe", "setupCommands": [ { "description": "Enable pretty-printing for gdb", "text": "-enable-pretty-printing", "ignoreFailures": true } ], "preLaunchTask": "C/C++: g++.exe build active file" } ] }
- program setting specifies the program you want to debug. set to the active file folder ${fileDirname} and active filename with the .exe extension ${fileBasenameNoExtension}.exe, which if helloworld.cpp is the active file will be helloworld.exe.
- By default, the C++ extension won’t add any breakpoints to your source code and the stopAtEntry value is set to false.
- Change the stopAtEntry value to true to cause the debugger to stop on the main method when you start debugging.
- The
preLaunchTask
setting is used to specify task to be executed before launch. Make sure it is consistent with thetasks.json
filelabel
setting
Start Debugging
To start debugging press F5 or from the main menu choose Run > Start Debugging.
The Integrated Terminal will appear at the bottom of the source code editor.
The editor highlights the first statement in the main method, a breakpoint that the C++ extension automatically sets
Step Through Code
The buttons include
Continue which runs until next breakpoint or completion
Step Over moves to the next line if there is no breakpoint in the code
Step Into moves to the next line of code
Step Out processes all code up to the next line of code outside of the function
Restart reloads code and starts a new debugging session
Stop exits the Debugging environment and code execution
Disconnect
breakpoints appear next to the line numbers.
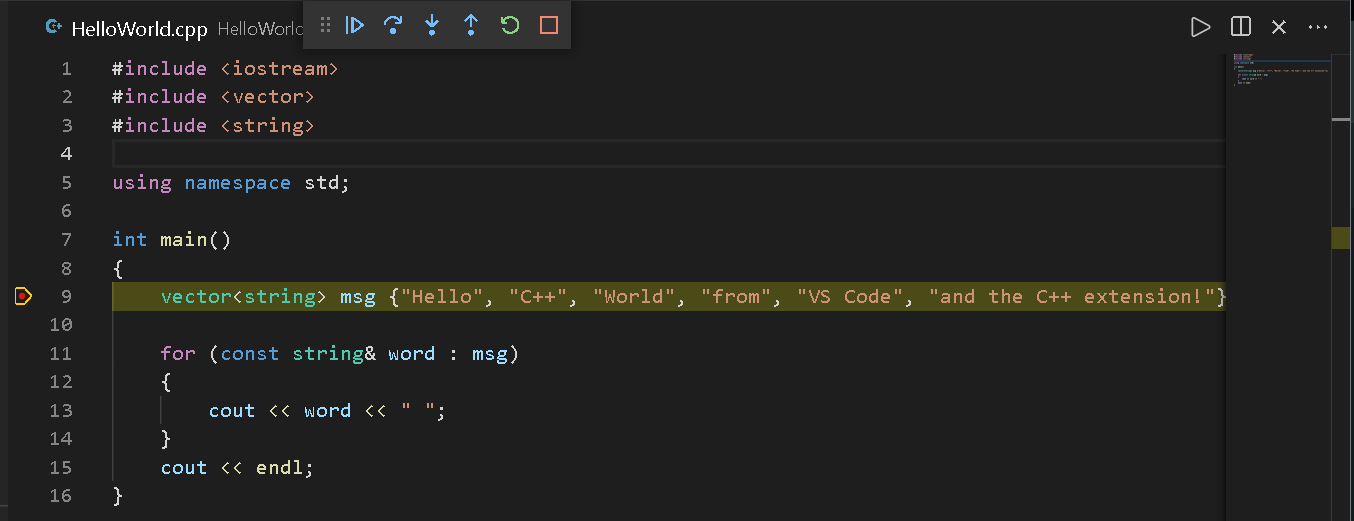
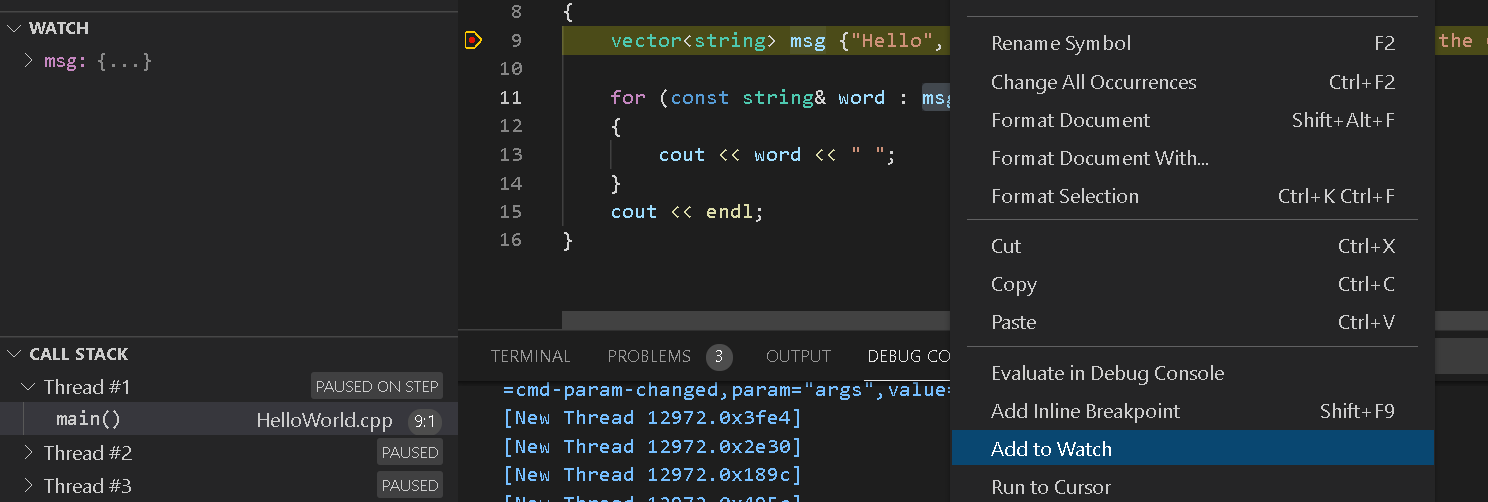
Creating Classes and using Classes in cpp files