Golang file server
Build a simple webserver with a home page, Hello, and a Function
Start by creating a Directory .\user\go\src\go-server and then open in VSCode
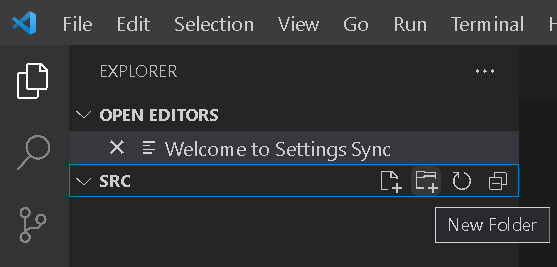
Add New File main.go
In main.go add code to setup server, The files in the Server will be setup as follows:
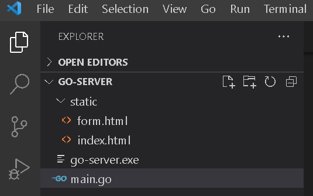
In main.go
Set up file package and import the libraries:
package main import ( "fmt" "log" "net/http" )
Add Functions
Add functions to handleFunc and handle. The handleFunc will process request for hello and form, the handle will process request with no handle
func formHandler(w http.ResponseWriter, r *http.Request) { if err := r.ParseForm(); err != nil { fmt.Fprintf(w, "ParseForm() err: %v", err) return } fmt.Fprintf(w, "POST request successful") name := r.FormValue("name") address := r.FormValue("address") fmt.Fprintf(w, "Name = %s\n", name) fmt.Fprintf(w, "Address = %s\n", address) } func helloHandler(w http.ResponseWriter, r *http.Request) { if r.URL.Path != "/hello" { http.Error(w, "404 not found", http.StatusNotFound) return } if r.Method != "GET" { http.Error(w, "method is not supported", http.StatusNotFound) return } fmt.Fprintf(w, "hello!") } func main() { fileserver := http.FileServer(http.Dir("./static")) http.Handle("/", fileserver) http.HandleFunc("/form", formHandler) http.HandleFunc("/hello", helloHandler) fmt.Printf("Starting server at port 8080\n") if err := http.ListenAndServe(":8080", nil); err != nil { log.Fatal(err) } }
Add Website html for serving requests
Add New Folder \static\ and Add two html files
Static html: will handle request to index.html
<!DOCTYPE html> <html> <head> <title> Static Website </title> </head> <body> <h2> Static Website </h2> </body
form.html: will handle Post Request to form
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"/> </head> <body> <Div> <form method="POST" action="/form"> <label>Name</label><input name="name" type="text" value=""/> <label>Address</label><input name="address" type="text" value=""/> <input type="submit" value="submit"/> </Div> </body> </html>
Hello.html will be generated by handleFunc
Start FileServer
on the terminal window type go build to create the binary file to execute
once the binary file is created run the file with go main.go
View/Dowload a copy of FilesServer design in PowerPoint